6.2.9 - SUI Options
What is options UI element?
An options UI element is like a list where you can choose an option from it. In SUI it's declared as SOptions.
We can add it to a panel or container as usual and we need to use the .Options method to specify the list of available options (passing an array of strings):
static string[] options = { "Option 1", "Option 2", "Option 3" };
public static void Create()
{
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Anchor(AnchorType.Fill)
- SOptions.Text("Options").Options(options);
panel.Add(container);
}
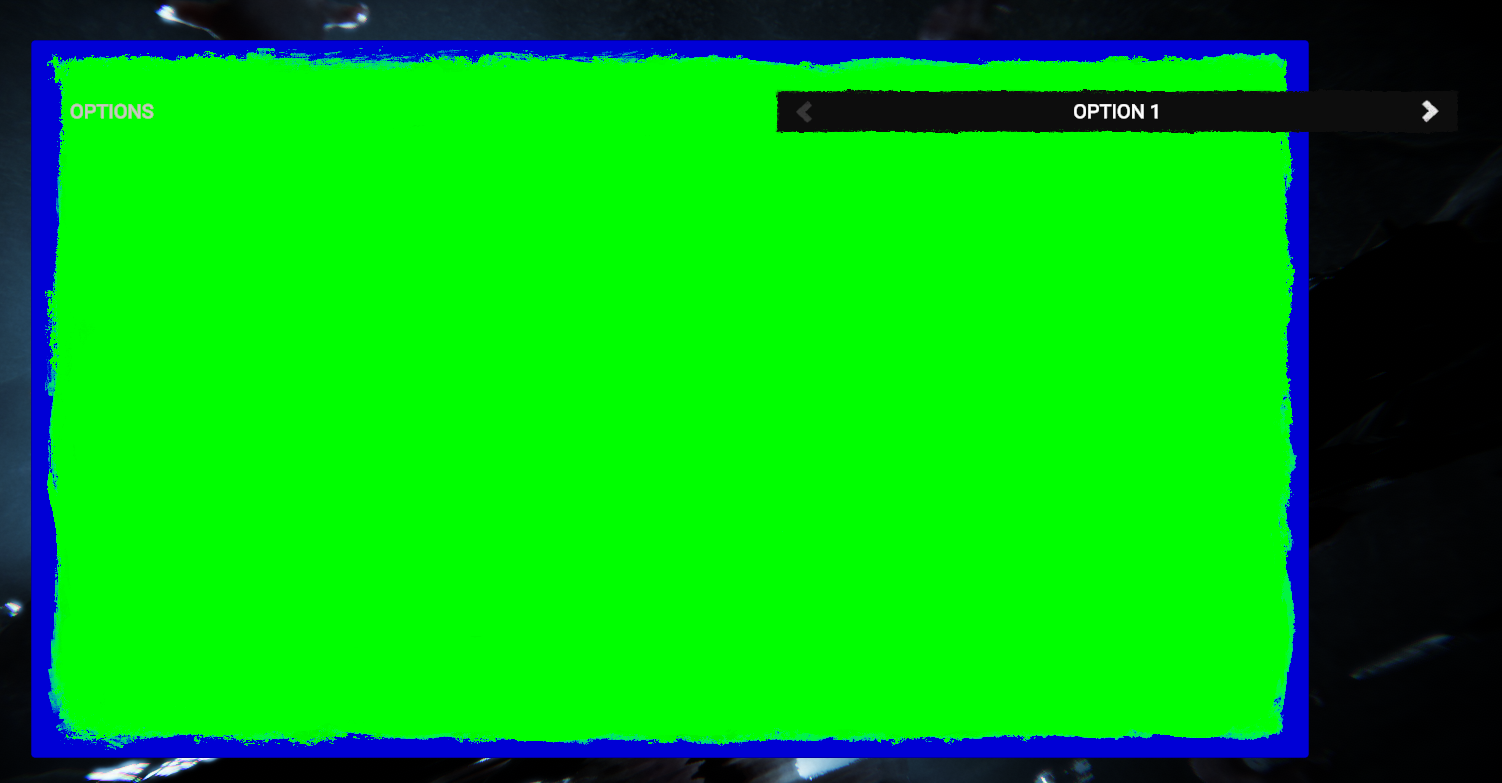
We can also do something when the selected option is changed using the .Notify method like so:
static string[] options = { "Option 1", "Option 2", "Option 3" };
public static void Create()
{
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Anchor(AnchorType.Fill)
- SOptions.Text("Options").Options(options).Notify(PrintOption); // passing the option index of the "options" array when changing the selection
panel.Add(container);
}
private static void PrintOption(int index) // receiving the index of the last selected option
{
switch (index)
{
case 0:
RLog.Msg("Option 1 selected");
break;
case 1:
RLog.Msg("Option 2 selected");
break;
case 2:
RLog.Msg("Option 3 selected");
break;
}
}